JavaScript 03
What is Page Redirection ?
You might have encountered a situation where you clicked a URL to reach a page X but internally you were directed to another page Y. It happens due to page redirection. This concept is different from JavaScript Page Refresh.
There could be various reasons why you would like to redirect a user from the original page. We are listing down a few of the reasons −
- You did not like the name of your domain and you are moving to a new one. In such a scenario, you may want to direct all your visitors to the new site. Here you can maintain your old domain but put a single page with a page redirection such that all your old domain visitors can come to your new domain.
- You have built-up various pages based on browser versions or their names or may be based on different countries, then instead of using your server-side page redirection, you can use client-side page redirection to land your users on the appropriate page.
- The Search Engines may have already indexed your pages. But while moving to another domain, you would not like to lose your visitors coming through search engines. So you can use client-side page redirection. But keep in mind this should not be done to fool the search engine, it could lead your site to get banned.
Alert Dialog Box
An alert dialog box is mostly used to give a warning message to the users. For example, if one input field requires to enter some text but the user does not provide any input, then as a part of validation, you can use an alert box to give a warning message.
Nonetheless, an alert box can still be used for friendlier messages. Alert box gives only one button "OK" to select and proceed.
Example
<html> <head> <script type = "text/javascript"> <!-- function Warn() { alert ("This is a warning message!"); document.write ("This is a warning message!"); } //--> </script> </head> <body> <p>Click the following button to see the result: </p> <form> <input type = "button" value = "Click Me" onclick = "Warn();" /> </form> </body> </html>
Confirmation Dialog Box
A confirmation dialog box is mostly used to take user's consent on any option. It displays a dialog box with two buttons: OK and Cancel.
If the user clicks on the OK button, the window method confirm() will return true. If the user clicks on the Cancel button, then confirm() returns false. You can use a confirmation dialog box as follows.
Example
<html> <head> <script type = "text/javascript"> <!-- function getConfirmation() { var retVal = confirm("Do you want to continue ?"); if( retVal == true ) { document.write ("User wants to continue!"); return true; } else { document.write ("User does not want to continue!"); return false; } } //--> </script> </head> <body> <p>Click the following button to see the result: </p> <form> <input type = "button" value = "Click Me" onclick = "getConfirmation();" /> </form> </body> </html>
Prompt Dialog Box
The prompt dialog box is very useful when you want to pop-up a text box to get user input. Thus, it enables you to interact with the user. The user needs to fill in the field and then click OK.
This dialog box is displayed using a method called prompt() which takes two parameters: (i) a label which you want to display in the text box and (ii) a default string to display in the text box.
This dialog box has two buttons: OK and Cancel. If the user clicks the OK button, the window method prompt() will return the entered value from the text box. If the user clicks the Cancel button, the window method prompt() returns null.
Example
The following example shows how to use a prompt dialog box −
<html> <head> <script type = "text/javascript"> <!-- function getValue() { var retVal = prompt("Enter your name : ", "your name here"); document.write("You have entered : " + retVal); } //--> </script> </head> <body> <p>Click the following button to see the result: </p> <form> <input type = "button" value = "Click Me" onclick = "getValue();" /> </form> </body> </html>
How to Print a Page?
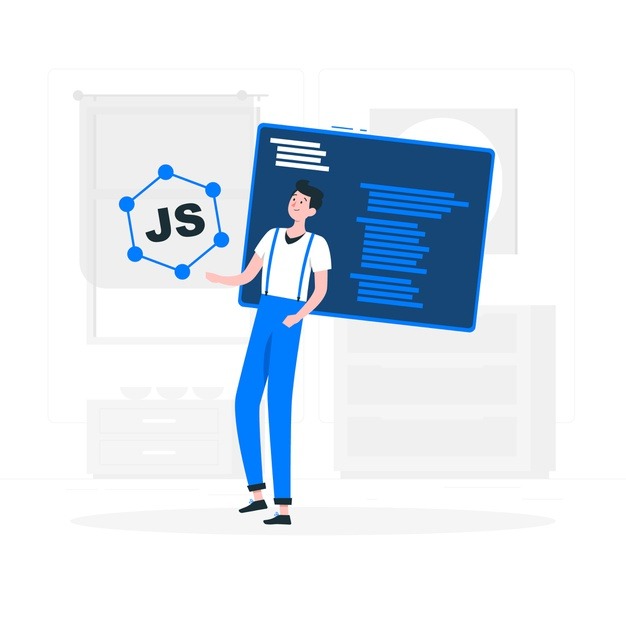
If you don’t find the above facilities on a web page, then you can use the browser's standard toolbar to get print the web page. Follow the link as follows.
File → Print → Click OK button.
Object Overview
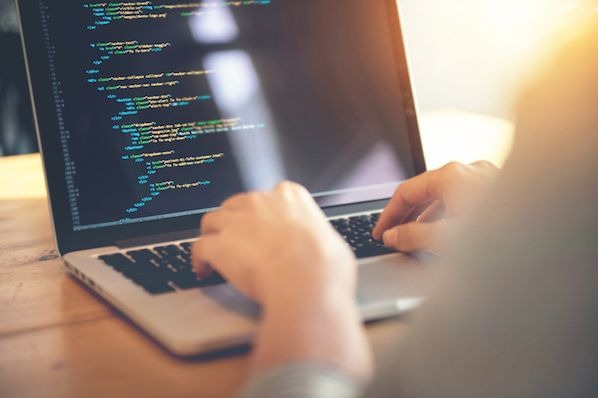
JavaScript is an Object Oriented Programming (OOP) language. A programming language can be called object-oriented if it provides four basic capabilities to developers −
- Encapsulation − the capability to store related information, whether data or methods, together in an object.
- Aggregation − the capability to store one object inside another object.
- Inheritance − the capability of a class to rely upon another class (or number of classes) for some of its properties and methods.
- Polymorphism − the capability to write one function or method that works in a variety of different ways.
Objects are composed of attributes. If an attribute contains a function, it is considered to be a method of the object, otherwise the attribute is considered a property.
Object Properties
Object properties can be any of the three primitive data types, or any of the abstract data types, such as another object. Object properties are usually variables that are used internally in the object's methods, but can also be globally visible variables that are used throughout the page.
The syntax for adding a property to an object is −
objectName.objectProperty = propertyValue;
For example − The following code gets the document title using the "title" property of the document object.
var str = document.title;
Object Methods
Methods are the functions that let the object do something or let something be done to it. There is a small difference between a function and a method – at a function is a standalone unit of statements and a method is attached to an object and can be referenced by the this keyword.
Methods are useful for everything from displaying the contents of the object to the screen to performing complex mathematical operations on a group of local properties and parameters.
For example − Following is a simple example to show how to use the write() method of document object to write any content on the document.
document.write("This is test");
User-Defined Objects
All user-defined objects and built-in objects are descendants of an object called Object.
The new Operator
The new operator is used to create an instance of an object. To create an object, the new operator is followed by the constructor method.
In the following example, the constructor methods are Object(), Array(), and Date(). These constructors are built-in JavaScript functions.
var employee = new Object(); var books = new Array("C++", "Perl", "Java"); var day = new Date("August 15, 1947");
The Object() Constructor
A constructor is a function that creates and initializes an object. JavaScript provides a special constructor function called Object() to build the object. The return value of the Object() constructor is assigned to a variable.
The variable contains a reference to the new object. The properties assigned to the object are not variables and are not defined with the var keyword.
Example 1
Try the following example; it demonstrates how to create an Object.
<html> <head> <title>User-defined objects</title> <script type = "text/javascript"> var book = new Object(); // Create the object book.subject = "Perl"; // Assign properties to the object book.author = "Mohtashim"; </script> </head> <body> <script type = "text/javascript"> document.write("Book name is : " + book.subject + "<br>"); document.write("Book author is : " + book.author + "<br>"); </script> </body> </html>
Output
Book name is : Perl Book author is : Mohtashim
Example 2
This example demonstrates how to create an object with a User-Defined Function. Here this keyword is used to refer to the object that has been passed to a function.
<html> <head> <title>User-defined objects</title> <script type = "text/javascript"> function book(title, author) { this.title = title; this.author = author; } </script> </head> <body> <script type = "text/javascript"> var myBook = new book("Perl", "Mohtashim"); document.write("Book title is : " + myBook.title + "<br>"); document.write("Book author is : " + myBook.author + "<br>"); </script> </body> </html>
Output
Book title is : Perl Book author is : Mohtashim
Defining Methods for an Object
The previous examples demonstrate how the constructor creates the object and assigns properties. But we need to complete the definition of an object by assigning methods to it.
Example
Try the following example; it shows how to add a function along with an object.
<html> <head> <title>User-defined objects</title> <script type = "text/javascript"> // Define a function which will work as a method function addPrice(amount) { this.price = amount; } function book(title, author) { this.title = title; this.author = author; this.addPrice = addPrice; // Assign that method as property. } </script> </head> <body> <script type = "text/javascript"> var myBook = new book("Perl", "Mohtashim"); myBook.addPrice(100); document.write("Book title is : " + myBook.title + "<br>"); document.write("Book author is : " + myBook.author + "<br>"); document.write("Book price is : " + myBook.price + "<br>"); </script> </body> </html>
The 'with' Keyword
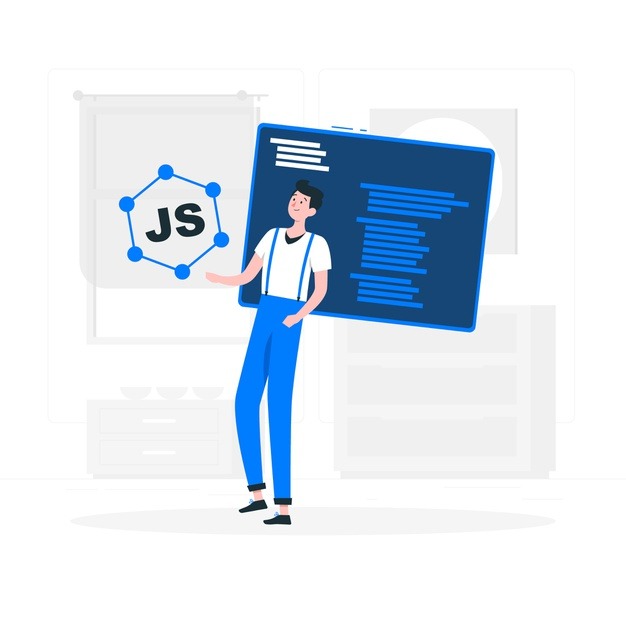
The ‘with’ keyword is used as a kind of shorthand for referencing an object's properties or methods.
The object specified as an argument to with becomes the default object for the duration of the block that follows. The properties and methods for the object can be used without naming the object.
Syntax
The syntax for with object is as follows −
with (object) { properties used without the object name and dot }
Comments
Post a Comment